There are a number of useful attributes in .NET that can be used to indicate to the C# compiler the null state of a particular variable.
They are split into 5 categories: Precondition, Postcondition, Conditional Postcondition, Method and property helper methods, and Unreachable Code.
Today, we're looking at the Precondition category that includes two Attributes, AllowNull
and DisallowNull
. Simply put, a precondition determines whether a parameter, field or property is allowed to be set to null.
The AllowNull
Attribute
The AllowNull
attribute can be used on a non-nullable parameter, field or property to indicate that whilst the property itself will never return null, it can accept null in order to set to a default value.
Imagine that we have a website that has a default theme and then users can pick their own theme. Our user entity may look something like this:
public class User
{
public string Name { get; set; }
public string EmailAddress { get; set; }
public Theme? Theme { get; set; }
}
Then we may have a view model to display a page.
public class BlogPageViewModel : PageViewModelBase
{
public DateTime CreatedAtUtc { get; set; }
}
And a base class for all of our page view models.
public abstract class PageViewModelBase
{
public Theme Theme { get; set; }
public string Title { get; set; }
}
When we create our view model, we have to check to see whether the user's theme is null and then get the default theme. However, surely that's code that should be in the view model. So we can use the AllowNull
attribute to tell the compiler that we're going to allow the setter to be called with a null value - this allows us to then replace this with a default value.
public abstract class PageViewModelBase
{
private Theme _theme = GetDefaultWebsiteTheme();
[AllowNull]
public Theme
{
get => _theme;
set => _theme = value ?? GetDefaultWebsiteTheme();
}
public string Title { get; set; }
}
This makes our code cleaner, as there is now only one place that needs to know about our default theme. If we pass a null value to the setter, the compiler won't generate a warning and the default value will be set.
The DisallowNull
Attribute
The DisallowNull
attribute works in reverse. It indicates that a nullable value cannot be set to null, although it may be null to start with.
private decimal? _price;
[DisallowNull]
public decimal? Price
{
get => _price;
set => _price = value ?? throw new ArgumentNullException(nameof(value), "Cannot set to null");
}
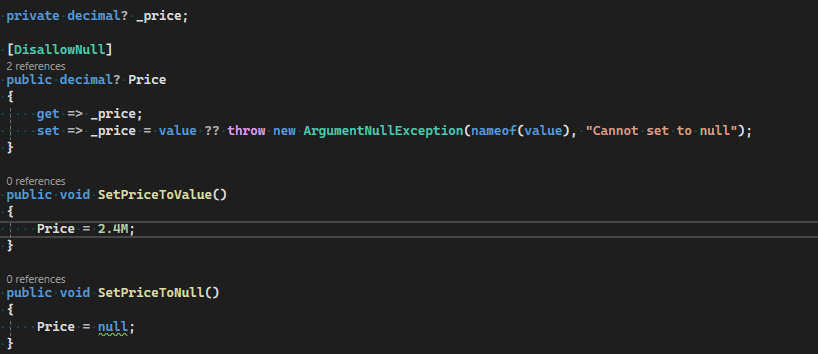
Summary
The Null-state Static Analysis Precondition attributes can help us make our code cleaner by indicating to the compiler as to whether a parameter, field or property is allowed to accept a null value or not.
More about the Precondition attributes can be found here.
Support me
If you liked this article then please consider supporting me.